Use Array in a While Loop PHP
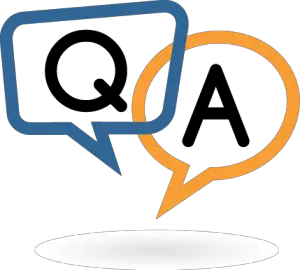
Â
Hello guys, please help me, I am confused about how to use array in a while loop, can you give me examples? Thanks
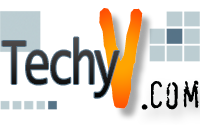
Â
Hello guys, please help me, I am confused about how to use array in a while loop, can you give me examples? Thanks
Hi Rhon,
Take a look at this code below. This is a very sample PHP code for using array in while loops.
<?php
   $number = array(1,2,3,4,5);
   $i = 5;
   while($i >= 0)
   {
      echo 'number['.$i.'] ='.$number[$i].'<br />';
      $i–;
   }
  Â
This is an example on how to access associative array using while loop.
   $losers['me'] = 1;
   $losers['you'] = 2;
   $losers['they'] = 3;
   echo 'losers<br />';
   while( $element = each( $losers ) )
   {
   echo $element[ 'key' ];
   echo ' – ';
   echo $element[ 'value' ];
   echo '<br />';
   }
// the other way around
   $winners[1] = 'me';
   $winners[2] = 'you';
   $winners[3] = 'they';
   echo 'winners<br />';
   while( $element = each( $winners ) )
   {
   echo $element[ 'key' ];
   echo ' – ';
   echo $element[ 'value' ];
   echo '<br />';
   }
?>