Programming uses algorithms regularly. They offer a set of guidelines for resolving various software issues. Developers’ lives can be made simpler by algorithms by providing them with various general problem-solving methods. There are currently thousands of programming algorithms available. Thus, good software engineers and developers must be aware of what is available and when it is best to apply it. A good algorithm will determine the fastest way to carry out a task or resolve a problem while also using the least amount of memory. In this article, we will discuss ten must-know algorithms for every programmer.
1. Binary Search Algorithm
One of the first concepts taught in each computer science course is binary search. It is possibly the simplest illustration of how a little creativity can make things immensely more effective. A binary search involves taking a sorted array, splitting it into two halves iteratively, and comparing an element you’re looking for to each half until you find it.
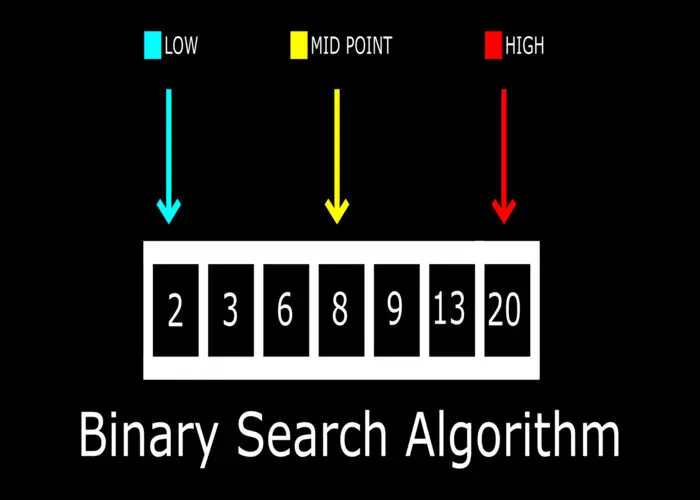
2. Merge Sort Algorithm
One of the most effective sorting algorithms is merge sort. Merge sort is a sorting method that divides an array into smaller subarrays, sorts each subarray, and then combines the sorted subarrays to create the final sorted array. The merge sort procedure involves splitting the array in half, sorting each half, and then joining the sorted halves back together. Up till the full array is sorted, this procedure is repeated.
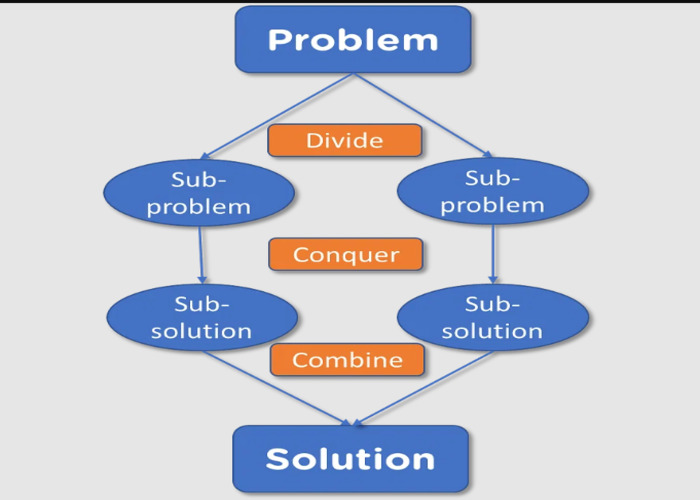
3. Quick Sort Algorithm
Quick sort is a very effective sorting method that works by dividing a large array of data into smaller arrays. An array is divided into two arrays, one of which contains values less than the pivot value—the value on which the division is made—and the other of which contains values higher than the pivot value.
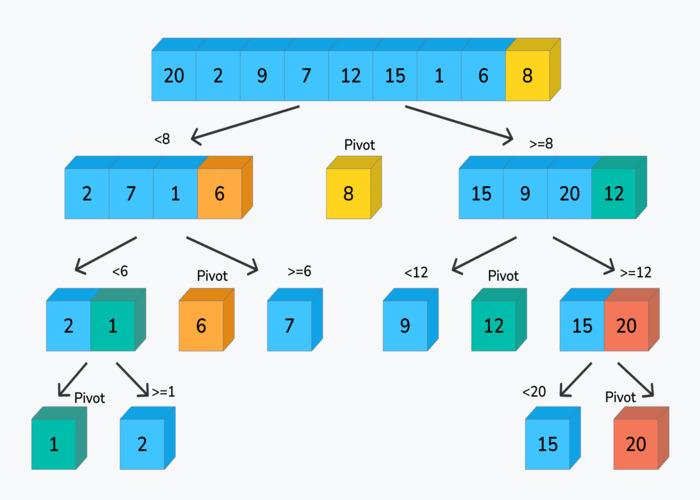
4. Breadth First Search Algorithm
The breadth-first search algorithm is a graph traversal algorithm that visits each node in a graph in breadth-first order, or all nodes at the current depth, before moving on to nodes at the next level. It functions by starting at a particular node, visiting its neighbors at that depth level, and then going on to the neighbors at the next depth level. The graph is visited at each node until all of them have been reached.
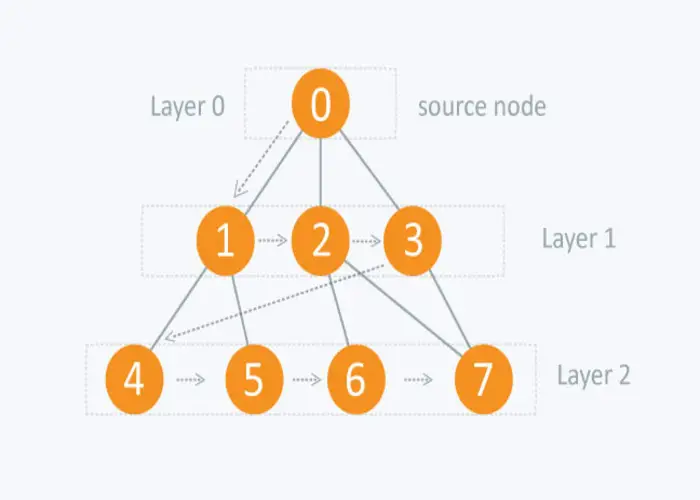
5. Depth First Search Algorithm
This algorithm is also a graph traversal method that visits each node in a graph in depth-first order, or as far as it can go before going back. Starting at a specific node, it proceeds to visit each branch as far as it can go before turning around to check the next branch. The graph is visited at each node until all of them have been reached.
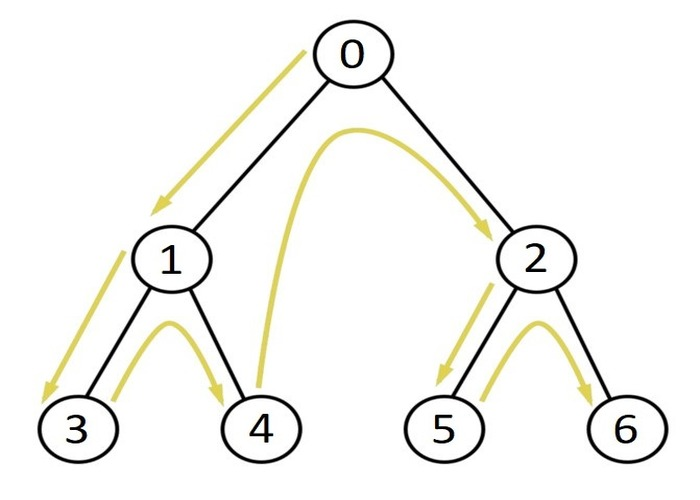
6. Dijkstra’s Algorithm
Dijkstra’s Algorithm is used to determine the shortest path between two nodes in a graph. You may create a shortest-path tree by determining the shortest path from a node (referred to as the “source node”) to every other node in the graph. To determine shortest path between the present location and the destination, GPS devices use this method. It has several industrial uses, particularly in fields where modeling networks are required.
7. Bellman Ford Algorithm
The Bellman Ford algorithm helps to find the shortest route across each vertex of a weighted graph. Although it is similar to Dijkstra’s algorithm, it is able to deal with graphs with edges that can have negative weights.
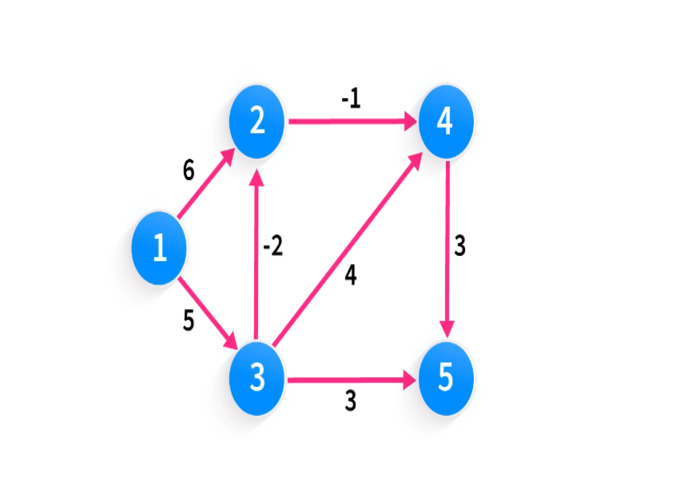
8. Knapsack Problem Algorithm
This dynamic programming algorithm resolves the knapsack problem, which includes selecting the subset of items with the highest value given a weight restriction. The Knapsack Problem is an optimization problem where we must select the best solution out of all feasible options.
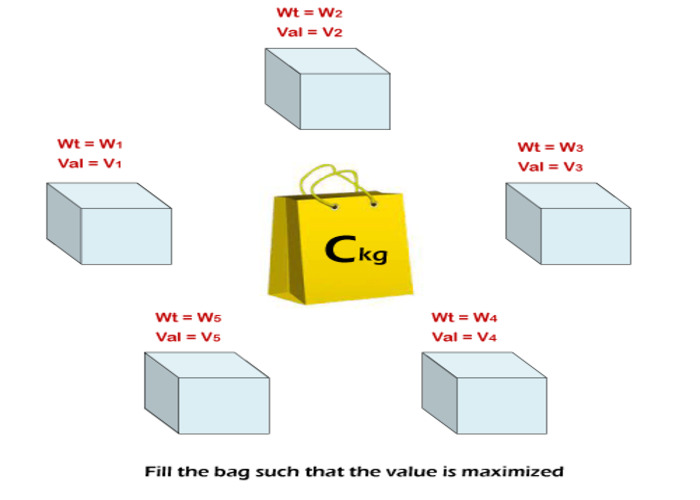
9. Kadane’s Algorithm
Kadane’s algorithm finds the maximum subarray sum of a given array of numbers. It functions by recording the maximum subarray sum found so far in this and the maximum subarray sum ending at each index. The algorithm updates the maximum subarray sum found so far, if necessary, by comparing the maximum subarray sum ending at each index with the maximum subarray sum ending at that index. The algorithm outputs the highest subarray sum that has been identified thus far.
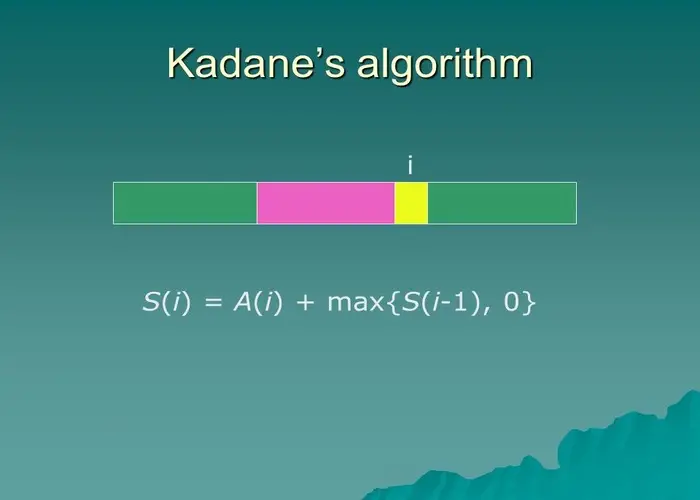
10. Hashing
A well-liked method for fast storing and retrieving data is hashing. Hashing is mainly used because it conducts optimal searches and gives optimal results. When keys and values are mapped into a hash table using a hash function, this process is known as hashing. For quicker access to the elements, it is done. The effectiveness of the hash algorithm used affects how efficiently data is mapped.
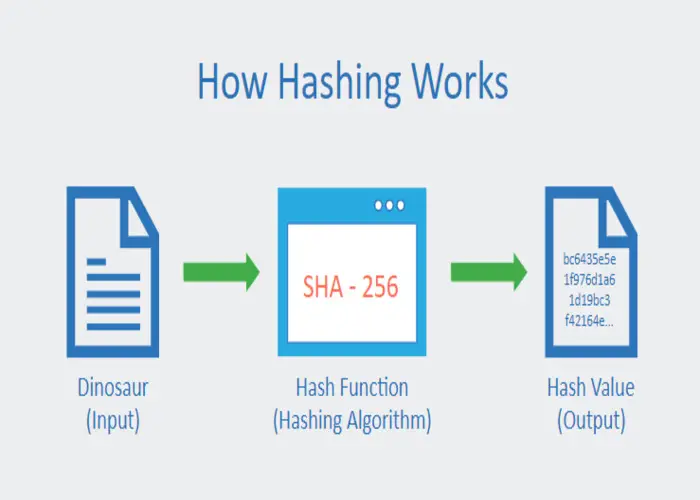