Basic PHP
Introduction
PHP, which stands for PHP: Hypertext Preprocessor. It was created by Rasmus Lerdorf in 1995 and originally expanded to Personal Home Page Tools. It was renamed to its current recursive form in version 3.0 when released in June 1998. Now , just the acronym PHP itself is widely known.
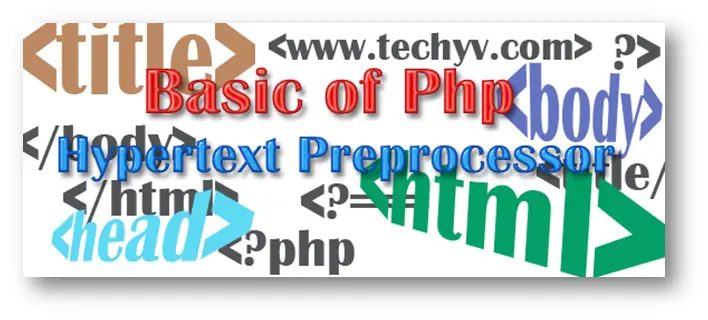
You might have wondered what is PHP and how it works after seeing the copious amount of opportunities for PHP in freelancing and other job sites. PHP is a server side scripting language used to design dynamic web pages, similar to ASP and JSP. Though HTML is used for the same purpose, much more can be done using PHP. You can collect data from users and have database interactions among many other things. Even large websites can be created very easily in a shorter amount of time.
PHP was designed to be used along with HTML. You can use PHP inside your existing HTML code, or you can put your HTML tags inside the PHP code. PHP is not an isolated language. It adds some functionality to an existing HTML code. And once the request is sent to server, the output produced will be a pure HTML document. If you right click on a page and click View Source (Internet Explorer), you won’t be able to find any evidence that the code contained PHP. The files with PHP coding would be saved with the extension PHP file.
PHP is mostly used along with MySQL database, but it can still be programmed to interact with any other database.
PHP Program
PHP code is always written within a PHP tag like this <?php ……..?>. It has a syntax very similar to that of a C/C++/Javascript. All statements end with a semicolon (;). All conditional statements and assignment statements are written in the same way. But variables can be declared same as in Javascript coding. The variables are un-typed and the variable names start with $.
Here is a sample PHP Program:
<html>
<head>
<title> My First PHP Page</title>
</head>
<body>
<?php
echo “Inside PHP”;
?>
</body>
</html>
The file with this code is to be saved with a.php extension. If it is placed on a PHP enabled server and loaded into the browser, it shows the output as Inside PHP. The first thing to be noted in the above sample is that the PHP code is written within a php tag and the php statement ends with a semicolon. The “echo” keyword is used to output a string. It is not actually a function, but a language construct.
Variables in PHP can be declared with a $ sign added at the beginning. They are also case sensitive. For example, if you plan to use a string welcome with a value, you should create it as: $welcome=”Hello Good Morning”. But for defining Constants, no dollar sign is needed. It can be done as follows:
define (“PI”, 3.14);
The data types available in PHP are integers, doubles and strings.
For a commenting on a single line in PHP there are 2 options –
1. //
2. #
For multiple line comments, start the commenting with /* and end it with */.
Different Operators available in PHP are
- Assignment Operators (=)
- Arithmetic Operators (+,-,*, /, %)
- Comparison Operators (==, =,<,>, ≤,≥)
- String Operators (.)
- Combination Arithmetic and Assignment Operators (+=,-=,*=, /=, %=,.=)
Different Statements available in PHP are
- If, if/else if statements
- Switch/case statement
- For, while and do while statements
- Include and require statements
All the above operators and statements would be familiar to any programmer with a basic programming knowledge; and thus there is no need to explain it in detail in this article. Include and require statements are identical and they are used to avoid code repetition. Suppose the same code would be repeated in many pages, that code can be kept in a single file and whenever it is required you can use an include statement with that particular file name. For example, if the repeating code is kept in a file named “common.php”, whenever you want to use that code you can specify <? php include (“common.php”); > or <? php require (“common.php”);>. You can thus avoid the repetition of a code. If an include statement comes across an error, it generates a warning but continue execution of the program. Meanwhile, when a require statement comes across an error, after generating the warning, it stops the execution of the program. The only difference between the two is in the way it handles an error.
Different Built-In Functions available are
- Array Manipulator Functions (merge, sort, count etc)
- Date and Time Functions (getdate, localtime, date etc)
- File system Functions (For dealing with flat files)
- Error Handling Functions
- Directory Functions
- Mail Functions (For dealing with e-mails)
- Database Functions ( For dealing with database)
PHP can also be used to handle many complex software requirements including but not limited to cookie handling, dynamic image handling, client server applications, encryption techniques, artificial intelligence and user interactions.
Advantages of PHP:
- Reduced Development Time: PHP helps to develop web applications very rapidly and efficiently.
- Speed: When compared with other scripting languages, PHP shows better performance in terms of speed. Even during complex processes like database interaction, it takes considerably less time. So it is commonly used in applications that need high performance e.g. server administration and mail functionalities among many others
- Platform independent: PHP supports many platforms like Windows, Linux etc. unlike other scripting languages that are designed for a particular platform.
- Easy syntax: Anybody who has a basic programming knowledge can easily learn the syntax of PHP. PHP is very much similar to C syntax.
- Database connectivity: PHP can be connected to a number of different databases.
- Open source: The user is given free license to reuse PHP thus making it an open source language
- PHP supports both structural and object oriented programming concepts.
- Easy deployment
Disadvantages of PHP:
- Error Handling: The main disadvantage of PHP is in the area of Error Handling. When it is compared to other scripting languages, PHP has relatively very poor error handling methods.
- Security flaws: As PHP is a web programming language; it is more prone to vulnerabilities.
- Performance: PHP does not provide performance for C or C++.
We should carefully consider the advantages and disadvantages of PHP as well as the requirements of our web application. It is our duty as programmers to make an informed decision on what would be the best choice of scripting languages for our web projects.