Object Oriented Programming Concepts
Different approaches are used in software development. Some of them are
- Procedural Oriented Programming
- Structured Programming (Modular Programming)
- Event driven Programming
- Object Oriented Programming
In Procedure Oriented Programming, the software is developed as a set of instructions which is executed one after another. But this method was inefficient while dealing with real time situations as the data and the procedures used to interact with this data were really separated. Structured Programming is based on a logical structure and is written in such a way that it can be modified easily. In Event driven Programming, the execution of the program is determined by some events.
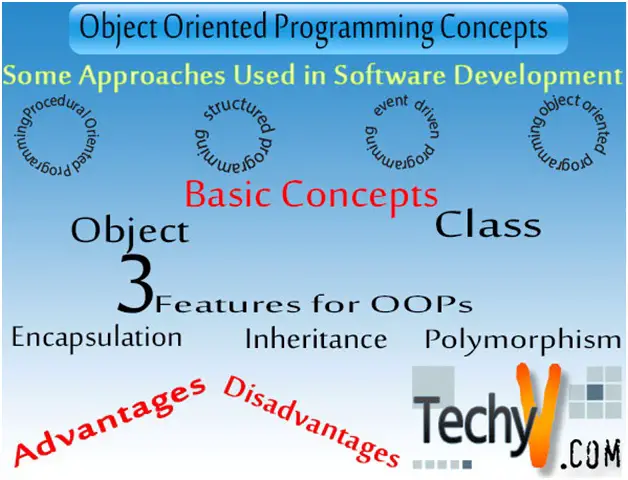
Among these 4 approaches, the one which is mostly used for implementing real time applications is Object Oriented Programming (OOPs).This is the reason why Object Oriented Programming are becoming so popular. As the name indicates, Object Oriented Programming is entirely based on objects. With the help of objects, real time system can be modeled. Anything in this world can be considered as an object with some characteristics. For e.g. car, bird, building etc. are actually real time objects. ADA, C++, Java, C# etc. are some of the commonly used Object Oriented Programming languages.
Basic Concepts
1. Object
An Object can be defined as a data structure consisting of some data fields and methods to operate on those data. Object does not have existence of own unless an instance of the class is created. Any number of objects can be created from a class.
2. Class
A Class is used to define an Object. For e.g. you define a Vehicle class. Then you could create Car and Bus as the objects of Vehicle class.
Code to implement Object and Class concepts using C++
The following code illustrates the concept of object and class Vehicle
class Vehicle
{
protected int noOfSeats, noOfWheels;
public void setParameters (int s,int w)
{
noOfSeats = s;
noOfWheels = w;
}
public int displayNoOfSeats()
{
return noOfSeats;
}
public int displayNoOfWheels
{
return noOfWheels;
}
};
int main ()
{
Vehicle car;
Vehicle cycle;
car. setParameters (5,4);
cycle. setParameters (1,2);
cout << car. displayNoOfSeats() << endl;
cout << cycle. displayNoOfWheels () << endl;
return 0;
}
In the above code Vehicle is a Class. Car and cycle are Objects. Here three common methods are defined in the class Vehicle. They are setParameters , displayNoOfSeats and displayNoOfWheels. setParameters method is used to set the values of number of wheels and number of seats to the object. The two remaining methods just return the no: of seats and no: of seats. For the car object the value for seats is set to 5 and the value for wheels is set to 4. In the same way the number of wheels for cycle is 2 and the number of seats for cycle is 1. And just to show that the display functions work properly, the count is called.
Features of Object Oriented Programming
Mainly there are 3 features for OOPs. They are
- Encapsulation
- Inheritance
- Polymorphism
Encapsulation is the process by which data hiding is made possible. Data and methods (functions used to access data) are combined into a single logical unit and access levels can be defined for this data and methods. Data and methods are together known as members. The three access levels are public, protected and private. Members which are defined as public can be accessed by all classes. Protected members can be accessed only within the same class and inherited classes. Private members can be accessed only within the same class. The above given code can be used to explain the concept of encapsulation. In the above example, the variables noOfSeats and noOfWheels can be accessed only within the same class and from the inherited class as they are defined as protected. And two other methods are defined to access these variables. Thus the data inside a class is not directly accessible. It can be accessed only using methods.
Inheritance is the process by which new classes can be created from the existing classes. The class from which the new classes are defined is known as Base class and the new classes created are known as derived classes. Code becomes reusable by inheritance. If you consider Shape as a base class, then Circle, Triangle and Rectangle can be considered as derived classes. That means Circle, Triangle and Rectangle have some common properties as that of Shape. Along with that some characteristics might be different. Inheritance can be defined to any level. It is also possible for a class to inherit from more than one class simultaneously. This is known as multiple inheritances. The syntax used for inheritance is : (full colon).
Polymorphism is the ability to exhibit different characteristics. Consider a method named add. The same method add can be used to add integers or even strings. For e.g. if you give 2 integers 5 and 4 as the input, then the result will be 9. If you give two strings “Good” and “Morning” as the input to this function, then the result will be “Good Morning”. This can be done with the help of polymorphism.
Advantages of OOPs
As OOP is based on the concept of objects which match the real world objects, the programming can be made simple and easy to handle. Simplicity is the main advantage of Object Oriented Programming. OOP provides high level of modularity. The data and the functions to access this data are combined into a single logical unit. Code reusability is easily made possible with the help of inheritance. If only some new features are to be added, a new class can be inherited from the existing class. Thus writing the entire code repeatedly again can be avoided. It is also easy to maintain the existing code. Thus maintainability is an added advantage.
Disadvantages of OOPs
The main problem with OOPs is that it is difficult to convert existing applications written in structured programming languages. Moreover, during execution it takes more time as it has to run many different routines.