ES6 or ECMAScript 2015 is the sixth version of the ECMAScript language specification standard. It set the standard for JavaScript implementation and became much more popular than the previous version of ES5. ES6 has brought significant changes to the JavaScript language. It brings several new features like let and const keywords, rest and spread operators, template characters, classes, modules, and many other improvements to make JavaScript programming easy and fun. We will discuss with you some of the best and most popular features of ES6 that we can use in writing your everyday JavaScript code.
1. Default Parameters
Default-valued function parameters are initialized with a default value if they contain no value or are undefined. JavaScript function parameters are initialized to undefined by default. However, it can be helpful to set a different default value. That is where the default settings come into play.

2. Destructuring
Developers can extract values from arrays and objects by deconstructing them and then exposing them as separate variables. Destructuring has a wide range of applications, including situations that require specific values from a collection. It provides a technique for quickly getting this value through built-in language features. The syntax of the deconstructed object with ‘{}’ and the syntax of the struct array with square brackets ‘[ ].’
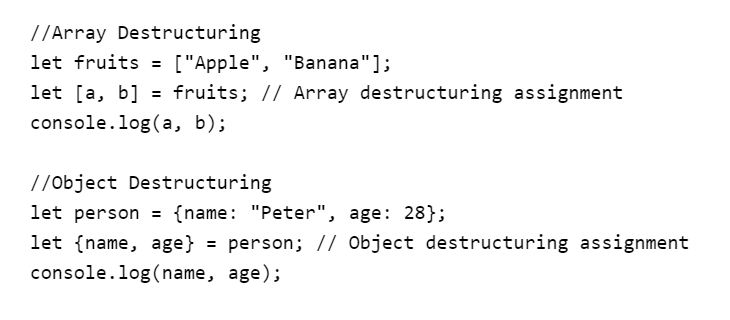
3. Const and Let Keyword
The keyword “let” allows the user to define variables, and on the other hand, “const” enables the user to define constants. Variables previously declared using “var” have function scope and are elevated to the top. That means a variable can be used before declaration. However, “let” variables and constants are block-scoped surrounded by curly braces “{}” and cannot be used before ordering.
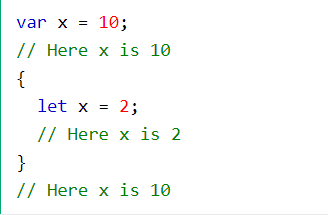
4. Arrow Function
ES6’s arrow functions give you another way to write shorter syntax than function expressions. The example defines a function that returns the sum of two numbers:
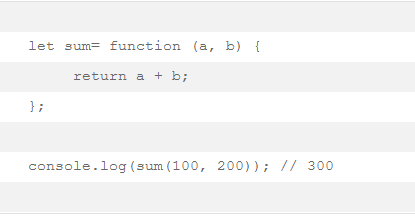
The following example is equivalent to the add() function but uses an arrow function instead:
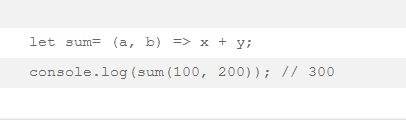
5. Template Literal
Template literal is a new feature introduced in ECMAScript 2015/ES6. It provides a straightforward way to create multiline strings and perform string interpolation. Pattern characters are string characters, and inline expressions are allowed. Before ES6, pattern characters were called template strings.

6. Multiline String
The new kid in town is named Template Strings. Template strings are delimited by a backtick (`) at each end and may contain additional backslashes if escaped with a backslash (i.e., let my_string =` some cool \ `hidden \` thing `). This new primitive type in JavaScript differs from string characters in several ways.
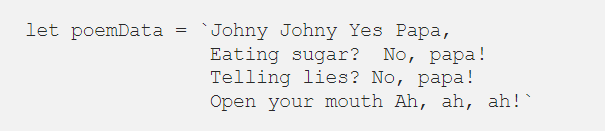
7. Enhanced Object Literals
Enhance object literal is used to group variables from the global scope and format them into javascript objects. That is the process of restructuring or repositioning. Object characters make it easy to quickly create things with properties inside curly braces.
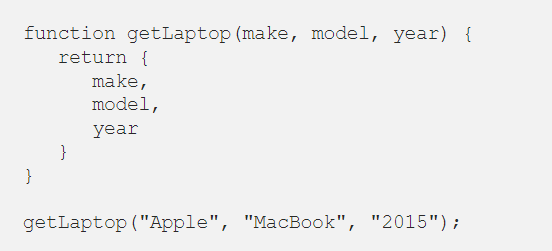
8. Classes
Classes have never existed in JavaScript. Classes were introduced in ES6 and are similar to classes in other object-oriented languages, such as C, Java, PHP, etc. But they don’t work the same way. ES6 classes simplify object creation, implement inheritance using the “expand” keyword, and efficiently reuse code.
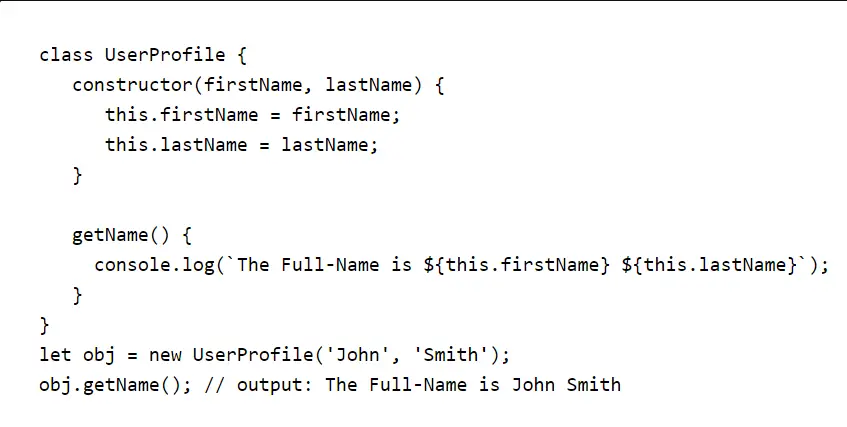
9. Modules
The module was previously not natively supported in JavaScript. The module is a new feature introduced in ES6, where each module is represented by a separate “.js” file. We can import or export variables, functions, classes, or any other components from/to different files and modules using the “import” or “export” declaration in a module.
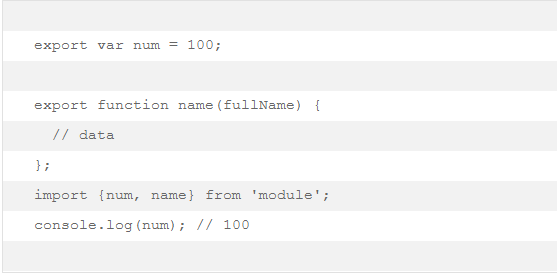
10. Promises
Promises are a clean way to implement asynchronous programming in JavaScript (a new ES6 feature). Before promises, callbacks were used to perform asynchronous programming. Let’s start by understanding what asynchronous programming is and how to implement it using Callbacks.
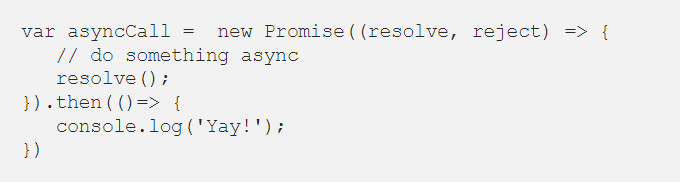