If you’re new to the world of programming and eager to learn the fundamentals of C++, you’re in the right place! In this guide, we’ll walk you through the top 10 simple C++ codes that are perfect for beginners. By learning these basic C++ codes, you’ll build a strong foundation and gain confidence in your programming skills. Let’s make one thing clear, you can only get hands-on programming by practising. So, practice as much as you can. Let’s start this exciting learning journey and discover the magic of C++!
1. Adding, Subtracting, Multiplying, Dividing And Calculating Power
First, you need to add the respective libraries.
#include <iostream>
//this library is necessary for almost all C++ programs as it is related to input and output
#include <cmath>
//this library has a lot of functions including the power function
using namespace std;
//for using standard names, functions and classes
Then, starting the main function we perform addition, subtraction, multiplication, and division on two numbers entered by the user. In the given example, I’ve calculated the square of both the user-entered numbers but you can use the pow() function to calculate any power.
system(“pause”);
return 0;
// should be added at the very end of every program so that the console pauses.
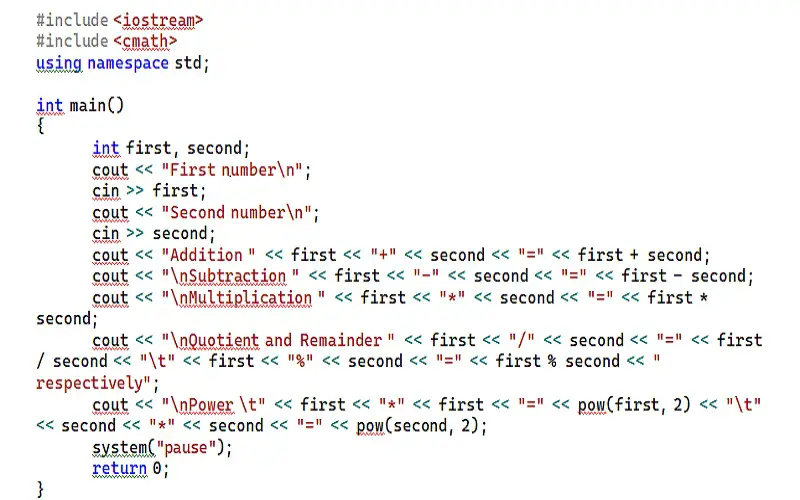
2. Displaying Prime Numbers
Here, we have displayed the prime numbers from 1 to 100. Like before, you have to add the basic necessary libraries for the program to run. For making any logic you have to write down a rough logic of what you want the output of the code to be and then make the logic accordingly. Prime numbers are those having only 2 factors (divisible only by themselves and 1). For this purpose, nested FOR loops are required. Keep a counter as well inside the loop.
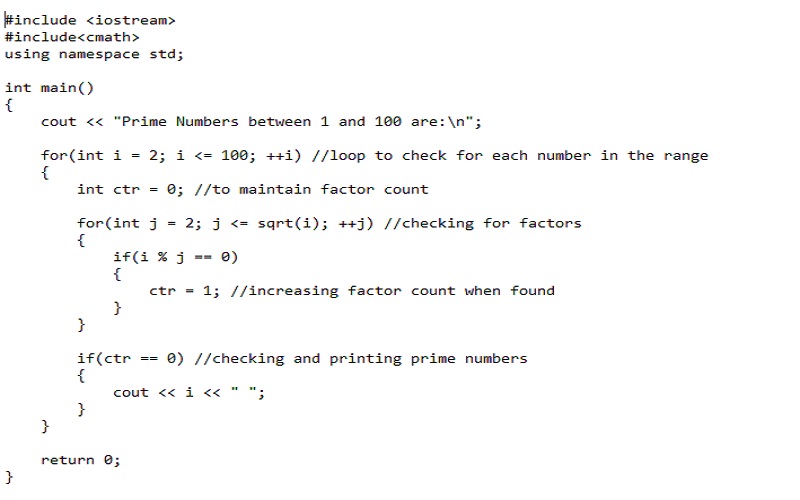
3. Swapping Values
For making this logic without declaring a third variable, we have to add the two numbers first in variable ‘a’. Now ‘a’ has the sum of both the variables then make the other variable ‘b’ equal to subtracting b from a. From this we will the value of ‘a’ that was formerly present. Now to get the previous value of ‘b’ in ‘a’ we have to subtract the present value of ‘b’ from the present value of ‘a’.
For example:
a=10 (actual value)
b=2 (actual value)
a=a+b=12 (new value)
b=a-b=10 (new swapped value)
a=a-b=2 (new swapped value)
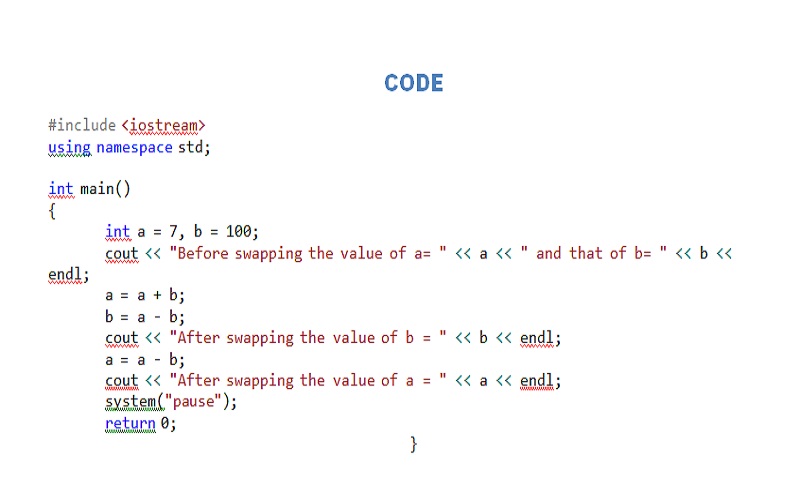
4. Finding The Largest Number
Here, we will be finding the largest number out of three user-entered numbers using if logic. You can also find the largest number out of more than 3 numbers by changing the logic according to the problem statement. Use of nested if loops is done in the given example.
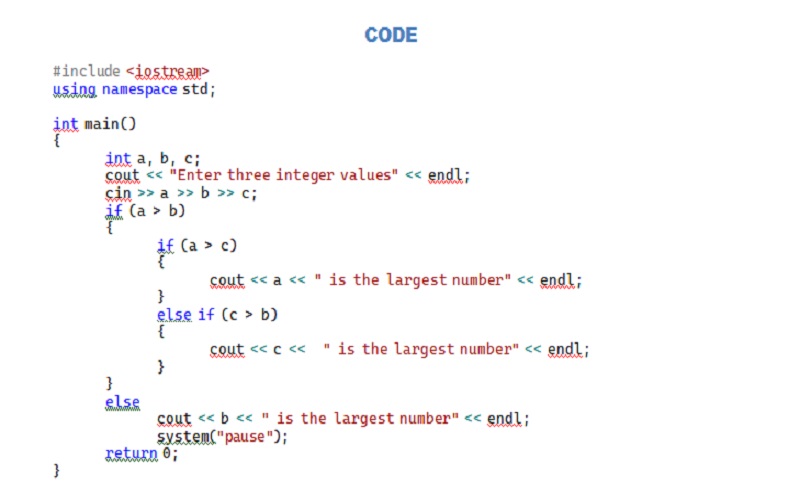
5. Fibonacci Sequence
Fibonacci Sequence is the one whose every next term is the sum of the previous two terms. So for this, the first two terms of a Fibonacci Sequence are always 0 and 1. Here, we will print the Fibonacci Sequence up to the integer entered by the user. For calculating the Fibonacci Sequence, WHILE loop is used in the following example.
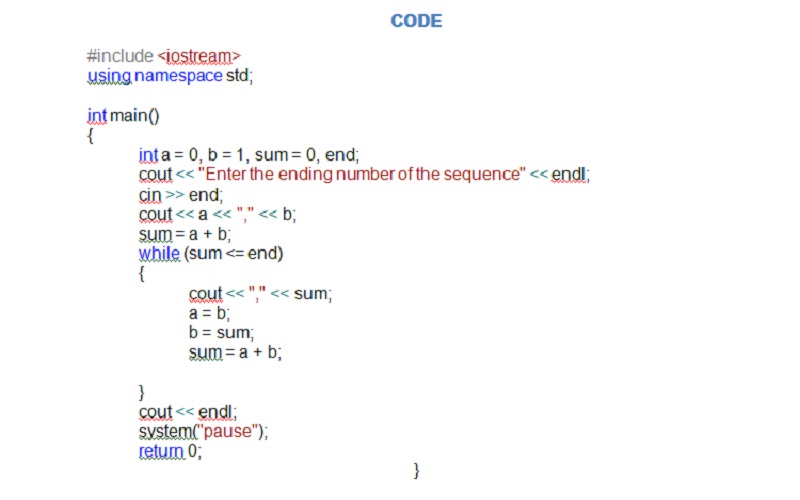
6. Identifying If An Alphabet Is A Vowel Or A Consonant
For this purpose, we have used case statements here and displayed vowel only if any one of the case statements is true else it will display consonant. The user first enters an alphabet and then the variable containing the alphabet is passed through the case statements and the correct answer is displayed.
If statement can also work for this problem. You can make any logic that suits the given situation.
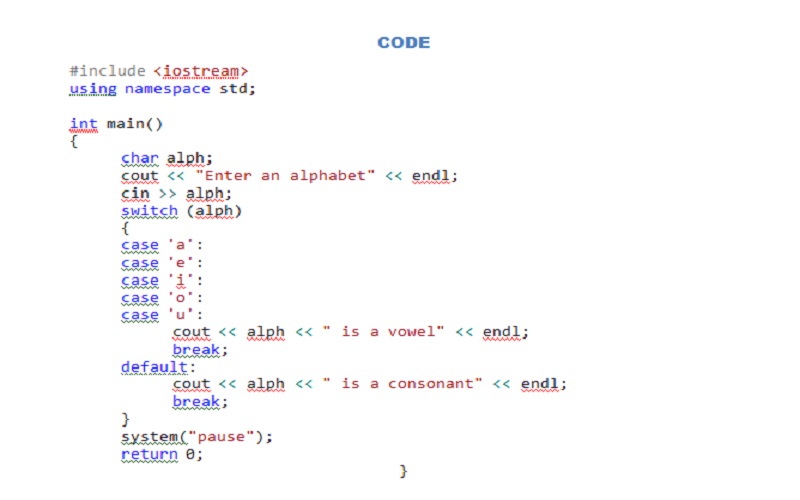
7. Printing The Table Of Any Integer
We can also print the table of any integer value entered by the user and can print the table up to any desired value. The example here is to print it up to 10. You can simply change the condition inside the for loop and print it to any value that fits the given statement.
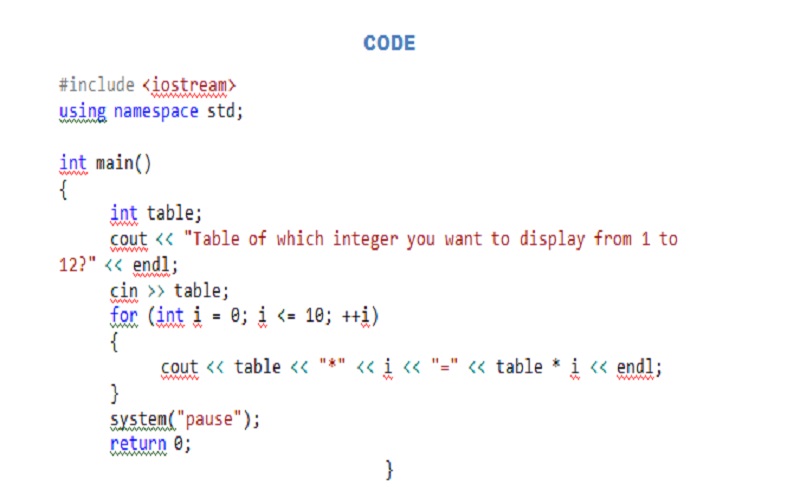
8. Calculating Factorial
Factorial is the multiplication from n to 1, where n could be any integer. For doing this in C++, we need to make a logic that includes a for loop that continues until the factorial is not calculated. In the given example, you can calculate the factorial of any number that is entered by the user.
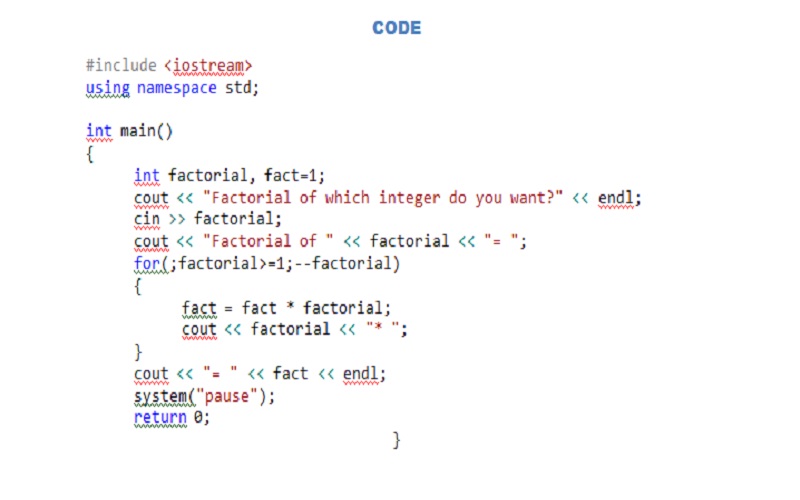
9. Printing String Backwards
For this purpose, first, we take input string from the user using the getline() function. In the given example, I have used the logic where first I save the size of the string in a variable and then print out the character present at the last position which is the size of the string. This works in a loop until the size is greater than or equal to zero.
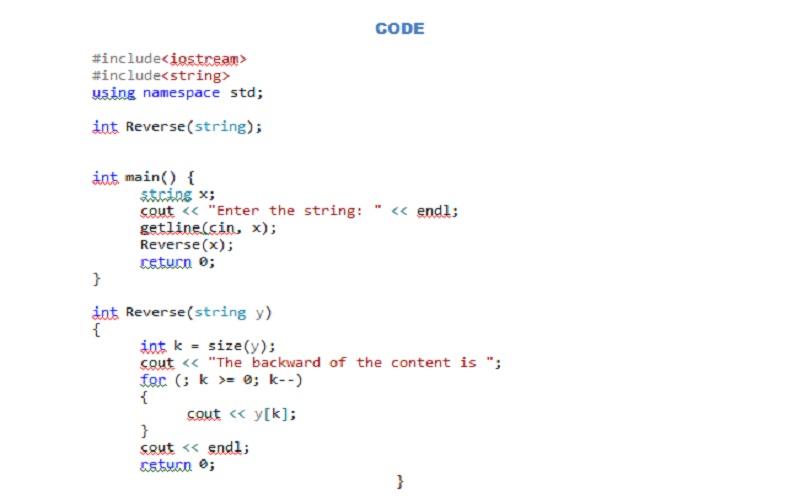
10. Finding The Length Of The String Using Pointers
The size of a string can be calculated using the size() function but what if we are asked to get the size of the string using pointers? Is that even possible? Oh, yes it is, I got you. Calculating that using pointers is quite easy. For that, you first need to do the same step which is to save the size of the variable in an integer and then refer the variable to a pointer.
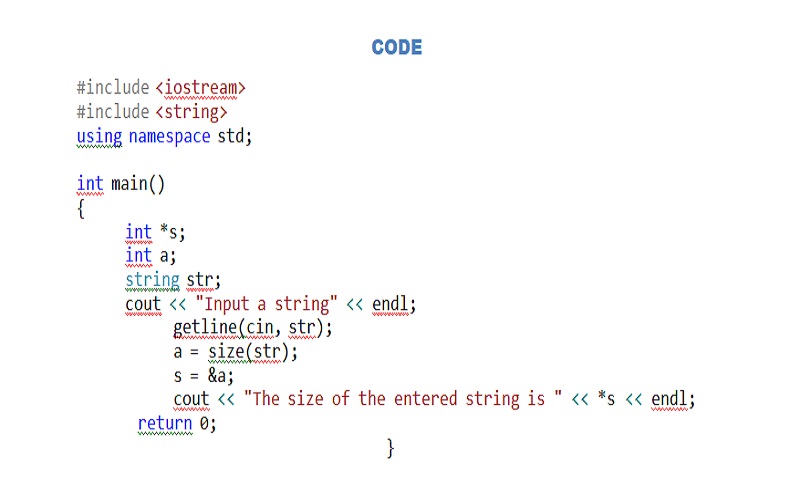