Calculate sum of first 1000 integers
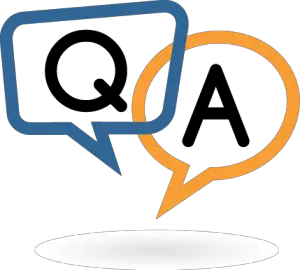
I need a program in C++ language for calculating the sum of first 1000 integers using while loop. And print the result.
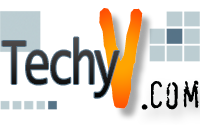
I need a program in C++ language for calculating the sum of first 1000 integers using while loop. And print the result.
Hi Eleanor,
You can try to use the sample program below:
include <iostream>
using namespace std;
int main()
{
// declarations of the variables
int sum,num;
//initialization of the variables
sum = 0;
num = 1;
//using the while loop to find out the sum of fist 1000 integers starting from one.
while number <= 1000)
{
//Adding the integers to the contents of sum
sum = sum + number;
//Generate the next integer by adding 1 to the integer
number = number + 1;
}
cout <<"The sum of the first 1000 integers starting from 1 is " <<sum;
}
Aristono
Let me make you clear that the previous answer to the question has only one problem. "num to be replaced by number.".
However I give you a fresh code.
#include < iostream.h >
void main ( ) {
int sum=0;Â Â Â Â Â Â Â Â Â Â Â //Initialize the sum variable
int ctr = 1;Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â // count for 1000 integers
while  ( ct r<= 1000 ){                     //check whether 1000 integers are done.
sum = sum + ctr;Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â //sum integer with the previous sum.
ctr = ctr + 1;Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â //increment to next integer
}
}
cout<<"Printing the result on the standard output : Sum is : Â "<<sum;Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â //Print the Summed result to standard output
In a more compact form
#inlcude < iostream.h >
void main ( ) {
int sum=0 , ctr = 1;           //Initialize the sum  and ctr variables
while  ( ct r<= 1000 )                     //check whether 1000 integers are done.
sum = sum + ctr++;Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â //sum integer with the previous sum and then increment.
cout<<"Printing the result on the standard output : Sum is : Â "<<sum;Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â //Print the Summed result to standard output
}
Â