Code for calculating circle Area and Circumference
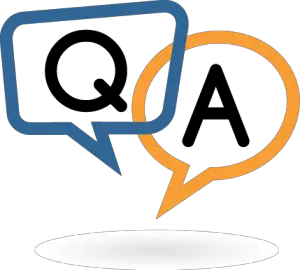
Need help with a program calculate the area and circumference of the circle by declaring a class
thanks in advance
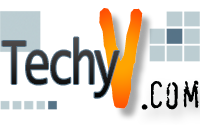
Need help with a program calculate the area and circumference of the circle by declaring a class
thanks in advance
//Â This program calculate the area and circumference of the circle by declaring a class
//Â Including header files in the program.
#include <iostream>
using namespace std;
// defining a macro for the value of PI
#define PI 3.14
//Â Beginning of the class Circle
class Circle {
  Â
  Â
   // declaring public members of the class
   public:
      Â
      double radius;   //   only data member of the class
     Â
      //      declaration of public member functions of the class
     Â
      Circle();           // default constructor
     Â
      Circle(double);      // Parameterized constructor
     Â
      double CalculateArea();      // calculates area of the circle and then returns
     Â
      double CalculateCircum();//      calculates circumference of the circle
     Â
      void Display();      //      displays the area and circumference of the screen
     Â
};
//Â Â Â Â Â Â end of class
//Â definition of default constructor of class
Circle::Circle()Â Â Â Â Â Â
{
   radius = 0.0;  // assignning default value to the data member radius
}
//Â Â Â definition of parameterized constructor of the class
Circle::Circle(double rad)
{
   radius = rad;
}
//Â Â Â definition of the function for area calculation of the circle
double Circle::CalculateArea()
{
   return (PI * radius * radius); // calculating area and then returning it the caller function
}
//Â Â Â definition of the function for calculating circumference of the circle
double Circle::CalculateCircum()
{
   return ( 2 * PI * radius ); // calculating and returning the circumferencr to the caller function
}
//Â Â Â definition of display() function
void Circle::Display()
{
  Â
   cout<<"nRadius of Circle:tt"<<radius;
   cout<<endl<<endl;
  Â
   cout<<"Area of Circle:ttt"<<CalculateArea();
   cout<<endl<<endl;
  Â
   cout<<"Circumference of Circlett"<<CalculateCircum();
   cout<<endl<<endl;  Â
}
//Â Â Â beginning of the main() function
main()
{
   Circle C1, C2(3.5);    // declaring two objects of class student
  Â
   //   for each object created, displaying the information on the screen
   C1.Display();  Â
   C2.Display();  Â
Â
   system("pause");   // system function to halt the output screen
}
Â
Check out this :
#include<iostream>
#include<cmath>
usingnamespacestd;
Â
intmain ()
{
Â
  floatradius;
  floatcircumference;
  floatarea;
Â
  cout <<"Please enter the radius of a circle: ";
  cin >>radius;
  cout <<"n";
Â
  circumference =2*3.1416*radius;
  area =3.1416*radius *radius;
Â
  cout <<"************************************"<<"n"
     <<"*Area and Circumference of A Circle*"<<"n"
     <<"************************************"<<"n"
     <<"tRadius= "<<radius <<"n"
     <<"tArea= "<<area <<"n"
     <<"tCircumference= "<<circumference <<"n";
Â
  cin.get();
Â
Â
  return0;
Â
}//end main
Â
Â
Â