Help on coding my Program
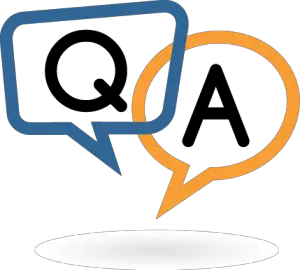
import java.awt.*; import javax.swing.* ; import java.awt.event.*; import java.net.*; import java.io.*; public class Client extends JApplet implements ActionListener, ItemListener { Container contentPane; JButton butcipher,butquit,butsend; JLabel lbl1,lbl2,lbl3,lbl4,lbl5,lbl6,lbl7; JRadioButton chksub,chktrans; ButtonGroup bgroup; JTextArea txtplanetxt,txtciphertxt; JTextField txtsubkey,txttranskey,txtipadd; JScrollPane jscrollpane1,jscrollpane2; String cryptmeth="Substitution"; String subciphertxt,transciphertxt,ptxt,subkeytxt,transkeytxt; int subkey=0; Socket sc; PrintStream out=null; BufferedReader in=null; public void init() { this.setSize(790,600); contentPane=getContentPane(); //obtain content pane contentPane.setBackground(new Color(135,162,252)); contentPane.setLayout(null); lbl6=new JLabel("Cryptography"); lbl6.setBounds(325,15,200,30); lbl6.setFont(new Font("Monotype Corsiva",Font.BOLD,30)); lbl6.setForeground(new Color(255,255,255)); contentPane.add(lbl6); lbl7=new JLabel("(Client)"); lbl7.setBounds(500,15,200,30); lbl7.setFont(new Font("",Font.BOLD,14)); contentPane.add(lbl7); lbl1=new JLabel("Enter Plane Text – "); lbl1.setBounds(75,40,200,30); lbl1.setFont(new Font("",Font.BOLD,14)); contentPane.add(lbl1); txtplanetxt=new JTextArea(5,25); JScrollPane jscrollpane1=new JScrollPane(txtplanetxt, ScrollPaneConstants.VERTICAL_SCROLLBAR_ALWAYS, ScrollPaneConstants.HORIZONTAL_SCROLLBAR_ALWAYS); jscrollpane1.setBounds(75,75,650,100); txtplanetxt.setFont(new Font("",Font.BOLD,14)); contentPane.add(jscrollpane1); chksub=new JRadioButton("Substitution Method", true); chksub.setBounds(170,190,200,30); chksub.setFont(new Font("",Font.BOLD,14)); contentPane.add(chksub); chksub.addItemListener(this); chktrans=new JRadioButton("Transposition Method"); chktrans.setBounds(480,190,200,30); chktrans.setFont(new Font("",Font.BOLD,14)); contentPane.add(chktrans); chktrans.addItemListener(this); bgroup=new ButtonGroup(); bgroup.add(chksub); bgroup.add(chktrans); lbl2=new JLabel("Enter Key – "); lbl2.setBounds(75,230,100,20); lbl2.setFont(new Font("",Font.BOLD,14)); contentPane.add(lbl2); txtsubkey=new JTextField(15); txtsubkey.setBounds(170,230,200,30); txtsubkey.setFont(new Font("",Font.BOLD,14)); contentPane.add(txtsubkey); lbl3=new JLabel("Enter Key – "); lbl3.setBounds(390,230,100,20); lbl3.setFont(new Font("",Font.BOLD,14)); contentPane.add(lbl3); txttranskey=new JTextField(15); txttranskey.setBounds(480,230,200,30); txttranskey.setFont(new Font("",Font.BOLD,14)); contentPane.add(txttranskey); lbl4=new JLabel("Cipher Text – "); lbl4.setBounds(75,270,200,30); lbl4.setFont(new Font("",Font.BOLD,14)); contentPane.add(lbl4); txtciphertxt=new JTextArea(5,25); txtciphertxt.enable(false); JScrollPane jscrollpane2=new JScrollPane(txtciphertxt, ScrollPaneConstants.VERTICAL_SCROLLBAR_ALWAYS, ScrollPaneConstants.HORIZONTAL_SCROLLBAR_ALWAYS); jscrollpane2.setBounds(75,300,650,100); txtciphertxt.setFont(new Font("",Font.BOLD,14)); contentPane.add(jscrollpane2); lbl5=new JLabel("Enter Server – "); lbl5.setBounds(230,420,200,20); lbl5.setFont(new Font("",Font.BOLD,14)); contentPane.add(lbl5); txtipadd=new JTextField(15); txtipadd.setBounds(390,420,150,30); txtipadd.setFont(new Font("",Font.BOLD,14)); contentPane.add(txtipadd); butcipher=new JButton("Cipher"); butcipher.setBounds(200,480,90,30); butcipher.addActionListener(this); contentPane.add(butcipher); butsend=new JButton("Send") ; butsend.setBounds(350,480,90,30); butsend.addActionListener(this); contentPane.add(butsend); butquit=new JButton("Quit") ; butquit.setBounds(500,480,90,30); butquit.addActionListener(this); contentPane.add(butquit); txtplanetxt.setFocusable(true); try { sc=new Socket("localhost",4444); System.out.println("Connected to server"); out = new PrintStream(sc.getOutputStream()); in = new BufferedReader(new InputStreamReader(sc.getInputStream())); } catch(Exception e) { System.out.print("Error :- " e); } } public void itemStateChanged(ItemEvent ie) { if(ie.getItemSelectable()==chksub) cryptmeth="Substitution"; if(ie.getItemSelectable()==chktrans) cryptmeth="Transposition"; } public void actionPerformed(ActionEvent ae) { if(ae.getActionCommand()=="Cipher") { txtciphertxt.setText(""); ptxt=new String(txtplanetxt.getText()); if(ptxt.length()<1) { JOptionPane.showMessageDialog((Component)null, "Please Enter Plain Text", "Input Message", JOptionPane.WARNING_MESSAGE); return ; } if(cryptmeth.equals("Substitution")) { subkeytxt=txtsubkey.getText(); try { subkey=Integer.parseInt(subkeytxt); if(subkey>=0
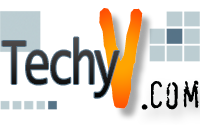