Need some login form help
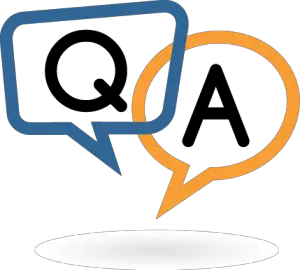
Hi everyone ,
I want to add user registration feature to my existing website so after the registration user must be able to login,
How can I have a database to store user names and passwords for each users?
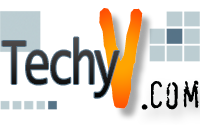
Hi everyone ,
I want to add user registration feature to my existing website so after the registration user must be able to login,
How can I have a database to store user names and passwords for each users?
First of all create the html page with name of login.php as below
<form action="login_process.php" method="POST">
USERNAME:<input type="text" name="name"><br />
Password:<input type="password" name="password" ><br />
<input type="submit" name="submit" value="LOG IN">
</form>
Then create the login_process.php page as below
<?php
include 'db_connect.php';
$name=$_POST['name'];
$password=$_POST['password'];
$sql="SELECT * FROM user_info WHERE user_name='$name'and password='$password'";
$result=mysql_query($sql);
$count=mysql_num_rows($result);
if($count==1)
{
header("location:newpost.php");
}
else{
echo "Wrong Username or Password";
}
?>
where db_connect.php page is
<?php
$a="localhost";
$b="root";
$c="";
$con = mysql_connect($a,$b,$c);
if(!$con)
{
die('Could not connect:' . mysql_error());
}
mysql_select_db("wblog", $con);
?>
where user_name and password are tables in the database wblog…
Hope you got the answer if you are smart enough or used php and mysql before..
P.S. if you get any trouble with this ..do inform me..
Thanks
<?php
Connection.php
<?php
Mysql_connect(“localhost”,”root”,””);
Mysql_select_db(“user”);
Here you have to give your database name.
?>
Registration.php
<?php
if(isset($_POST['submit']))
{
include("include/connection.php");
$a=$_POST["name"];
$b=$_POST["email"];
$c=$_POST["password"];
$d=$_POST["country"];
$e=$_POST["state"];
$query=mysql_query("insert into reg (name,email,password,country,state)
values ('”.$a.”','”.$b.”','”.$c.”','”.$d.”','”.$e.”')")or
die(mysql_error());
if($query)
{
echo "ok inserted";
}
else
{
echo "wrong";
}}
?>
This is the related html coding
<form action="" method="post"name="form">
<table width="200" border="0">
<tr>
<td>name:</td>
<td><label>
<input type="text"name="name">
</label></td>
</tr>
<tr>
<td>email:</td>
<td><label>
<input type="text"name="email">
</label></td>
</tr>
<tr>
<td>password:</td>
<td><label>
<input type="password"name="password">
</label></td>
</tr>
<tr>
<td>country:</td>
<td><label>
<select name="country">
<option>india </option>
<option>pakistan </option>
<option>china </option>
<option>
russia </option>
<option>england </option>
<option>bhutan </option>
<option>
nepal </option>
</select>
</label></td>
</tr>
<tr>
<td>state:</td>
<td><label>
<select name="state">
<option>
haryana </option>
<option>
bihar </option>
</select>
</label></td>
</tr>
<tr>
<td>
</td><td>
<input type="submit"name="submit" value="Submit">
</label></td>
</tr>
<tr>
<td> </td>
</tr>
</table></form>
Login.php
<formaction="login_check.php"method="POST">
USERNAME:<input type="text"name="name"><br />
Password:<input type="password"name="password" ><br />
<input type="submit" name="submit" value="LOG IN">
</form>
Then create the
login_check.php page as below
<?php
include 'connectionphp';
$name=$_POST['name'];
$password=$_POST['password'];
$sql="SELECT * FROM reg WHERE name='$name'and password='$password'";
$result=mysql_query($sql);
$count=mysql_num_rows($result);
if($count==1)
{
echo “login successfully”;
}
else{
echo "Wrong Username or Password";
}
?>
Notifications