Problems with Linux programming looping examples
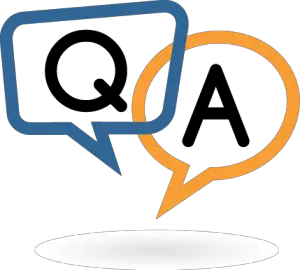
After learning the basics of programming shell scripts in Linux, I am having trouble with looping and am looking for some examples of how to perform this function.
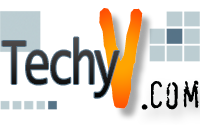
After learning the basics of programming shell scripts in Linux, I am having trouble with looping and am looking for some examples of how to perform this function.
Just like 'C' Language looping Implementation,its same in the Shell Scripting with the While,If-Else,For.In every case a block is executed repeatedly until an exit condition is satisfied.
Syntax IF
if[ condition ] then
fi
For ex-
write a program for odd and even
echo"Enter the no"
read a
if[ a%2 -ge 0 ]
     echo "even"
else
      echo "odd"
fi
//As You can see a is a variable.in the if condition -ge stands for greater than or equal to.i.e.if a is equal to 0,its even otherwise odd.
Syntax while
while[ cond. ]
do
done
for ex.
echo "Enter the number"
read num
fact=1
while[ $num -gt 0 ]
do
let fact=fact*num;
let num=num-1
done
echo"Factorial value of num is"
echo $fact
//fact is a variable that has value i.num is user defined variable.this loop executes till num is greater than 1.suppose num is 5.it is greater than 0.
1 iteration-Â Â Â Â Â Â Â fact=1*5
                            num-5-1
2 Iteration:-Â Â Â Â Â Â Â num is 4 and greater than 0.so cond true.loop executes.fact=5
                            fact=5*4
                            num=4-1
3 Iteration:Â Â Â Â Â Â Â Â Â num is now 3>0.cond true.loop executes.fact=20
                             fact=20*3
                             num=3-1
4 Iteration:Â Â Â Â Â Â Â Â Â num is now 2>0.loop executes.fact=60
                            fact=60*2
                            num=2-1
5 Iteration:Â Â Â Â Â Â Â Â Â num is now 1>0.loop executes.fact=120
                            fact=120*1
                            num=1-1
6 Iteration          num is now 0.is is not greater than 0.condition false.loop break.
                            Answer is 120.
Syntax Nested If-else
if[ condition ]
else if[ condition ]
else
fi
fi
for ex:
WAP to find the greatest no of three nos.
echo "Enter three nos"
read a,b,c
if[ a -gt b ]then
        if[ c -gt a ] then
               echo $ b
else if
               echo $c
fi
fi
fi
These are operators
-eq            equal to
-gt               greater than
-ge             greater than or equal to
-lt                less than
-le               less than or equal to
-ne              not equal to
Â