Want to create a registration form PHP
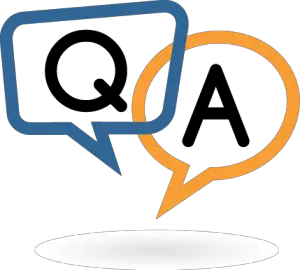
Dear Experts
I am interested in creating a registration form/sign up form for a website. Can any expert tell me to write the script for best php registration form? I have worked a lot but couldn’t succeed.
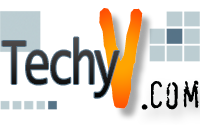
Dear Experts
I am interested in creating a registration form/sign up form for a website. Can any expert tell me to write the script for best php registration form? I have worked a lot but couldn’t succeed.
Hi Nood
I think having a registration form is a must if you are planning to have a dynamic website. Here is a tutorial that is 100% working.
Simple Registration Form Using PHP and MySQL Here are the things that you’ll learn in that tutorial:
I hope this helps.
The very first thing to do to create a registration form in PHP is to create a new MySQL database and then insert a table containing information like username, userid, and password.
CREATE TABLE `users` (
`userid` INT( 11 ) NOT NULL AUTO_INCREMENT PRIMARY KEY ,
`username` VARCHAR( 50 ) NOT NULL ,
`password` VARCHAR( 50 ) NOT NULL ,
UNIQUE (
`username`
)
) ENGINE = MYISAM ;
The next step is to create the actual registration form in PHP called “registration.php”.
<html>
<head>
<title>Registration form</title>
</head>
<body>
<form method="post" action="check.php" enctype="multipart/form-data">
<table width="300" border="0">
<tr>
<td>Username:</td>
<td><input type="text" name="username"></td>
</tr>
<tr>
<td>Password:</td>
<td><input type="password" name="password"></td>
</tr>
<span style="size:10%;color:#FF0000"><?php if(isset($_GET["pass"])) { echo $_GET["pass"]; } ?></span>
<tr>
<td> </td>
<td><input type="submit" value="Sing up" name="registration" /></td>
</tr>
</table>
</form>
</body>
</html>
The output should be similar to this:
After this, you need to create a connection to the database called “connection.php”.
<?
$name = "root";
$pas = "google";
$dbname = "helpinghands";
$con = mysql_connect("localhost",$name,$pas);
mysql_select_db($dbname,$con);
?>
Lastly, you need to create a check file called “check.php”. This will do the actual registration process.
<?php
if(isset($_POST['registration']))
{
require "connection.php";
$username = strip_tags($_POST['username']);
$password = md5(strip_tags($_POST['password']));
mysql_query("INSERT INTO users(username,password) VALUES ('$username','$password')")
or die("".mysql_error());
echo "Successful Registration!";
}
?>
Make one database named "mydb".
Create one table named "registration" in phpmyadmin.
CREATE TABLE `registration` (
`user_noi` int(11) NOT NULL auto_increment,
`user_namev` varchar(50) collate latin1_general_ci NOT NULL default '',
`user_sexv` varchar(6) collate latin1_general_ci NOT NULL default '',
`user_date_of_birthd` date NOT NULL default '0000-00-00',
`user_agei` int(11) NOT NULL default '0',
`user_countryi` int(11) NOT NULL default '0',
`user_statei` int(11) NOT NULL default '0',
`user_cityi` int(11) NOT NULL default '0',
`user_hightv` varchar(10) collate latin1_general_ci NOT NULL default '',
`user_marital_statusv` varchar(10) collate latin1_general_ci NOT NULL default '',
`user_email_idv` varchar(30) collate latin1_general_ci NOT NULL default '',
`user_idv` varchar(30) collate latin1_general_ci NOT NULL default '',
`user_passwordv` varchar(30) collate latin1_general_ci NOT NULL default '',
`user_phonenov` varchar(20) collate latin1_general_ci NOT NULL default '',
`user_mobile_nov` varchar(12) collate latin1_general_ci NOT NULL default '',
`user_profile_postedv` varchar(10) collate latin1_general_ci NOT NULL default '',PRIMARY KEY (`user_noi`)
) ENGINE=MyISAM DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci AUTO_INCREMENT=11 ;
It will Look like this page.
Now make one file named "config.php" to connect with localhost
<?php
$servername='localhost';
$dbusername='root';
$dbpassword='';
$dbname='mydb';
connecttodb($servername,$dbname,$dbusername,$dbpassword);
function connecttodb($servername,$dbname,$dbuser,$dbpassword)
{
global $conn;
$conn=@mysql_connect ($servername,$dbuser,$dbpassword);
if(!$conn)
{
header("location:errorpage.html");
}
mysql_select_db("$dbname",$conn);
if(mysql_error())
{
echo "connection error";
}
}
?>
Here is designed HTMl form for registering a user.
Make one file named "register.php".
Put this code in that file.
<?php include_once("config.php");
$msg="";
if(isset($_POST['txtuserid'])&& $_POST['txtuserid']!="")
{
$q1=mysql_query("select * from tbluser_account_detail where user_idv='".$_POST['txtuserid']."'");
//echo $q1;
$r=mysql_num_rows($q1);
if($r==0)
{
if(isset($_POST['txtusername'])&&$_POST['txtusername']!="")
{
$dt=$_POST['lstyear']."-".$_POST['lstmonth']."-".$_POST['lstday']; // concatnation of date
$age=date("Y")-($_POST['lstyear']);
$q="INSERT INTO tbluser_account_detail(user_namev,user_sexv,user_date_of_birthd,user_countryi,user_statei,user_cityi,
user_hightv,user_marital_statusv,user_email_idv,user_idv,user_passwordv,user_phonenov,user_mobile_nov,user_profile_postedv,user_agei)
VALUES ('".$_POST['txtusername']."','".$_POST['optsex']."','".$dt."',".$_POST['lstcountry'].",".$_POST['lststate'].",".$_POST['lstcity'].",'".$_POST['lstheight']."','".$_POST['optmaritalstatus']."','".$_POST['txtemail']."','".$_POST['txtuserid']."','".$_POST['txtpassword']."','".$_POST['txttelephoneno']."','".$_POST['txtmobileno']."','".$_POST['optprofile']."',".$age.")";
mysql_query($q);
echo " record inserted";
}
}
else
{
$msg="User id is also used by other user";
}
}
?>
<link href="include/style.css" rel="stylesheet" type="text/css" />
<script language="javascript">
function chkrdetail()
{
if(document.registeruser.txtusername.value=="")
{
alert("Enter The User Name ");
document.registeruser.txtusername.focus();
return false;
}
else if(document.registeruser.lstday.value=="")
{
alert("Select The Day ");
document.registeruser.lstday.focus();
return false;
}
else if(document.registeruser.lstmonth.value=="")
{
alert("Select The Month ");
document.registeruser.lstmonth.focus();
return false;
}
else if(document.registeruser.lstyear.value=="")
{
alert("Select The Year ");
document.registeruser.lstyear.focus();
return false;
}
else if(document.registeruser.lstcountry.value=="")
{
alert("Select The Country ");
document.registeruser.lstcountry.focus();
return false;
}
else if(document.registeruser.lststate.value=="")
{
alert("Select The State ");
document.registeruser.lststate.focus();
return false;
}
else if(document.registeruser.lstcity.value=="")
{
alert("Select The City ");
document.registeruser.lstcity.focus();
return false;
}
else if(document.registeruser.lstheight.value=="")
{
alert("Select The Height ");
document.registeruser.lstheight.focus();
return false;
}
else if(document.registeruser.txtemail.value=="")
{
alert("Enter The Email Address ");
document.registeruser.txtemail.focus();
return false;
}
else if(document.registeruser.txtuseid.value=="")
{
alert("Enter The Used Id ");
document.registeruser.txtuseid.focus();
return false;
}
else if(document.registeruser.txtpassword.value=="")
{
alert("Enter The Passsword");
document.registeruser.txtpassword.focus();
return false;
}
else if(document.registeruser.txtconpassword.value=="")
{
alert("Enter The Passsword");
document.registeruser.txtconpassword.focus();
return false;
}
else if(document.registeruser.txtconpassword.value!=document.registeruser.txtpassword.value)
{
alert("Choose password and confrim password not match");
document.registeruser.txtpassword.focus();
return false;
}
else if(document.registeruser.lstmtongue.value=="")
{
alert("Select The Mother Tongue ");
document.registeruser.lstmtongue.focus();
return false;
}
else if(document.registeruser.lstreligion.value=="")
{
alert("Select The Religion ");
document.registeruser.lstreligion.focus();
return false;
}
else if(document.registeruser.lstcaste.value=="")
{
alert("Select The Caste ");
document.registeruser.lstcaste.focus();
return false;
}
else if(document.registeruser.txtabout.value=="")
{
alert("Enter About your Self ");
document.registeruser.txtabout.focus();
return false;
}
else
{
return true;
}
return false;
}
</script>
<form action="" method="post" name="registeruser">
<br />
<table><tr><td align="center" colspan="2"><?php=$msg;?></td></tr></table>
<table width="780" border="1" class="table_border" align="center">
<tr><td colspan="2" align="center"><h1 class="heding" >Account Details</h1></td></tr>
<tr>
<td class="labels">*Your Name:</td>
<td ><input type="text" name="txtusername" class="textinputcolor"></td>
</tr>
<tr>
<td class="labels">*Gender:</td>
<td class="textinputcolor">
<input name="optsex" type="radio" value="Male" checked="checked" >Male
<input name="optsex" type="radio" value="Female"> Female
</td>
</tr>
<tr>
<td class="labels">* Date of Birth</td>
<td><select name="lstday" class="textinputcolor">
<option value="" selected="selected"> Day </option>
<option value="01">1</option>
<option value="02">2</option>
<option value="03">3</option>
<option value="04">4</option>
<option value="05">5</option>
<option value="06">6</option>
<option value="07">7</option>
<option value="08">8</option>
<option value="09">9</option>
<option value="10">10</option>
<option value="11">11</option>
<option value="12">12</option>
<option value="13">13</option>
<option value="14">14</option>
<option value="15">15</option>
<option value="16">16</option>
<option value="17">17</option>
<option value="18">18</option>
<option value="19">19</option>
<option value="20">20</option>
<option value="21">21</option>
<option value="22">22</option>
<option value="23">23</option>
<option value="24">24</option>
<option value="25">25</option>
<option value="26">26</option>
<option value="27">27</option>
<option value="28">28</option>
<option value="29">29</option>
<option value="30">30</option>
<option value="31">31</option>
</select>
<select name="lstmonth" class="textinputcolor">
<option value="" selected="selected"> Month </option>
<option value="01">January</option>
<option value="02">Feburuary</option>
<option value="03">March</option>
<option value="04">April</option>
<option value="05">May</option>
<option value="06">June</option>
<option value="07">July</option>
<option value="08">August</option>
<option value="09">September</option>
<option value="10">October</option>
<option value="11">November</option>
<option value="12">December</option>
</select>
<select name="lstyear" class="textinputcolor">
<option value="" selected="selected"> Year </option>
<?php for($i=1975;$i<=2099;$i++)
echo "<option value=".$i.">".$i."</option>";
?>
</select>
</td>
</tr>
<tr>
<td class="labels">*Country</td>
<td ><select name="lstcountry" class="textinputcolor"><option value="" selected="selected">Select The Country </option>
<option value="India">India</option>
<option value="Japan">Us</option>
</select></td>
</tr>
<tr>
<td class="labels">*State</td>
<td ><select name="lststate" class="textinputcolor"><option value="" selected="selected">Select The state </option>
<option>Gujarat</option>
<option>Rajasthan</option>
<option>Maharashtra</option>
</select></td>
</tr>
<tr>
<td class="labels">*City</td>
<td ><select name="lstcity" class="textinputcolor"><option value="" selected="selected">Select The City </option>
<option>Surat</option>
<option>Bombay</option>
<option>Navsari</option>
</select></td>
</tr>
<tr>
<td class="labels">* Height:</td>
<td>
<select name="lstheight" class="textinputcolor">
<option value="" selected="selected">Select The Height</option>
<option value="4-05">4' 5" (1.35 mts)</option>
<option value="4-06">4' 6" (1.37 mts)</option>
<option value="4-07">4' 7" (1.40 mts)</option>
<option value="4-08">4' 8" (1.42 mts)</option>
<option value="4-09">4' 9" (1.45 mts)</option>
<option value="4-10">4' 10" (1.47 mts)</option>
<option value="4-11">4' 11" (1.50 mts)</option>
<option value="5-00">5' 0" (1.52 mts)</option>
<option value="5-01">5' 1" (1.55 mts)</option>
<option value="5-02">5' 2" (1.58 mts)</option>
<option value="5-03">5' 3" (1.60 mts)</option>
<option value="5-04">5' 4" (1.63 mts)</option>
<option value="5-05">5' 5" (1.65 mts)</option>
<option value="5-06">5' 6" (1.68 mts)</option>
<option value="5-07">5' 7" (1.70 mts)</option>
<option value="5-08">5' 8" (1.73 mts)</option>
<option value="5-09">5' 9" (1.75 mts)</option>
<option value="5-10">5' 10" (1.78 mts)</option>
<option value="5-11">5' 11" (1.80 mts)</option>
<option value="6-00">6' 0" (1.83 mts)</option>
<option value="6-01">6' 1" (1.85 mts)</option>
<option value="6-02">6' 2" (1.88 mts)</option>
<option value="6-03">6' 3" (1.91 mts)</option>
<option value="6-04">6' 4" (1.93 mts)</option>
<option value="6-05">6' 5" (1.96 mts)</option>
<option value="6-06">6' 6" (1.98 mts)</option>
<option value="6-07">6' 7" (2.01 mts)</option>
<option value="6-08">6' 8" (2.03 mts)</option>
<option value="6-09">6' 9" (2.06 mts)</option>
<option value="6-10">6' 10" (2.08 mts)</option>
<option value="6-11">6' 11" (2.11 mts)</option>
<option value="7-00">7' (2.13 mts) plus</option>
</select>
</td>
</tr>
<tr>
<td class="labels">* Marital Status:</td>
<td class="textinputcolor"><input name="optmaritalstatus" type="radio" value="Single" checked="checked">Single
<input name="optmaritalstatus" type="radio" value="Widowed">Widowed
<input name="optmaritalstatus" type="radio" value="Divorcee">Divorcee
<input name="optmaritalstatus" type="radio" value="Annulled">Annulled
</td>
</tr>
<tr>
<td class="labels">* Email:</td>
<td><input type="text" name="txtemail" class="textinputcolor"></td>
</tr>
<tr>
<td width="196" class="labels">* User Id </td>
<td width="568" ><input type="text" name="txtuserid" class="textinputcolor"></td>
</tr>
<tr>
<td class="labels">* Choose Password:</td>
<td><input type="password" name="txtpassword" class="textinputcolor"></td>
</tr>
<tr>
<td class="labels">* Confrim Password:</td>
<td><input type="password" name="txtconpassword" class="textinputcolor"></td>
</tr>
<tr>
<td class="labels"> Telephone Number:</td>
<td><input type="text" name="txttelephoneno" class="textinputcolor"></td>
</tr>
<tr>
<td class="labels">Mobile Number:</td>
<td><input type="text" name="txtmobileno" class="textinputcolor"></td>
</tr>
<tr>
<td class="labels">* Profile Posted by:</td>
<td class="textinputcolor"><input name="optprofile" type="radio" value="Self" checked="checked">Self
<input name="optprofile" type="radio" value="Parents, Guardian">Parents, Guardian
<input name="optprofile" type="radio" value="Sibling">Sibling
<input name="optprofile" type="radio" value="Friend">Friend
<input name="optprofile" type="radio" value="Marriage Bureau">Marriage Bureau
<input name="optprofile" type="radio" value="Others">Others</td>
</tr>
</table>
<table class="table_border" align="center">
<tr>
<td align="center" colspan="2"><input name="image" type="image" src="images/exigo_finish.jpg" onclick="return chkrdetail();"/></td>
</tr>
</table>
</table>
</form>
<script type="text/javascript">
document.registeruser.txtusername.focus();
</script>