What is Recursion or Recursive Function in Terms of Programming?
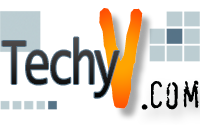
Recursion is the ability to a procedure to call itself. It is easy to use recursion rather than using iteration in some cases such as adding up squares and the most famous one is finding a factorial.
The ability of a procedure to call itself is referred to as Recursion. Most of the programming languages allow recursion and in some case it is used without using the iterations and loops because it is more efficiency and less utilization of memory.
Thanks Akash ED and Asiri. Are there any examples with you? If so please be good enough to send me a one.
You are welcome Hemantha. Check the following two examples given.
Recursive solution for adding up squares
Int SumSquares(int m, int n)
{
   if(m < n )
                return m*m + SumSquares(m+1,n);  // Calling the function itself
   else
                return m*m ;
}Â Â Â Â Â Â Â Â
Recursive solution for calculating the Factorial
Int factorial(int n)
{
   if( n==1 )
                return 1;
   else
                return n * factorial(n-1) ; //Calling the same function
}
The most famous application of recursion is calculating factorial.
Calculating Factorial of 6
factorial(6)Â = (6*factorial(5))
                         = (6*(5*factorial(4)))
                         = (6*(5*(4*factorial(3))))
                               = (6*(5*(4*(3*factorial(2)))))
                               = (6*(5*(4*(3*(2*factorial(1))))))
                               = (6*(5*(4*(3*(2*1)))))
                               = (6*(5*(4*(3*2))))
                               = (6*(5*(4*6)))
                               = (6*(5*24))
                               = (6*120)
                               = 720
Recursive Factorial Method
Public long factorial(long number)
{
   if( number<=1 )
                return 1;
   else
                return number * factorial(number-1) ;
}Â Â Â